SQL Injection in the Age of ORM: Risks, Mitigations, and Best Practices
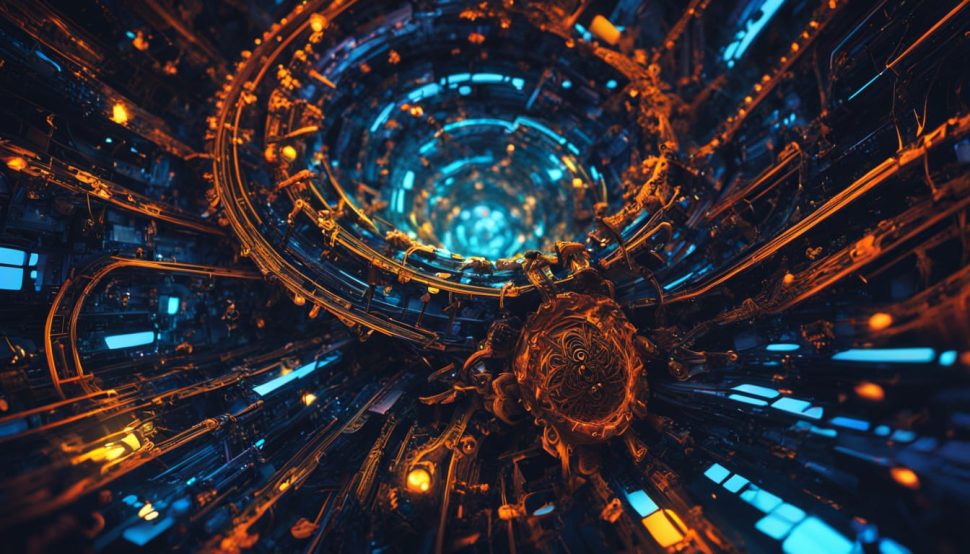
SQL injection remains one of the most dangerous and widespread vulnerabilities in modern web applications. To guard against these attacks, many developers rely on Object-Relational Mapping (ORM) frameworks, assuming that abstracting away from raw SQL queries inherently provides protection. However, this sense of security can be deceptive. While ORM frameworks offer strong safeguards against SQL injection, they are not a silver bullet. Improper implementation or flaws within the frameworks themselves can still introduce vulnerabilities.
Enjoy!
Understanding ORM and Its Security Implications
Object-Relational Mapping (ORM) frameworks serve as intermediaries between application code and databases, allowing developers to work with database records as objects in their programming language of choice. By abstracting away direct SQL queries, ORMs can help prevent the composition errors that often lead to SQL injection vulnerabilities. However, just because developers aren’t manually writing SQL doesn’t mean SQL isn’t being generated behind the scenes.
The False Sense of Security
Many developers assume that using an ORM framework automatically protects their applications from SQL injection attacks. This assumption creates a dangerous false sense of security. As noted in security research, several popular ORM packages have themselves contained SQL injection vulnerabilities, including four vulnerabilities reported in the top npm ORM packages, Sequelize and node-mysql. These vulnerabilities existed within the frameworks themselves, demonstrating that no layer of abstraction is immune to security flaws.
ORM frameworks essentially translate object operations into SQL commands. If the framework itself contains flaws in how it constructs these SQL commands, or if developers use certain features incorrectly, SQL injection vulnerabilities can still emerge.
This reality shifts the security focus from “ORM prevents SQL injection” to “ORM can help prevent SQL injection when used correctly.”
How SQL Injection Persists in ORM-Based Applications
SQL injection vulnerabilities can still manifest in ORM-based applications through several pathways, despite the abstraction and safety mechanisms these frameworks provide:
- Mixing raw SQL with ORM operations: Developers sometimes resort to raw SQL for complex queries, but if user input is improperly handled in these cases, the application becomes vulnerable.
- Improper use of ORM features that bypass parameterization: Manually constructing queries with string concatenation instead of using the built-in parameter binding mechanisms can lead to injection flaws.
- Vulnerabilities within the ORM framework itself: Like any software, ORMs can have their own bugs or edge cases that expose applications to risk.
- Using outdated versions of ORM frameworks with known vulnerabilities: Failing to keep dependencies up to date may leave the application exposed to already-patched security issues.
The persistence of these vulnerabilities highlights that using an ORM is not a guarantee of safety. Developers must maintain a security-conscious mindset and follow best practices consistently, even when working with higher-level abstractions.
Proper training, code reviews, and security testing remain critical components of secure software development.
Vulnerable ORM Implementations Across Technologies
To understand the practical implications of SQL injection vulnerabilities in applications using ORM frameworks, it’s important to look at how these issues can appear in real-world code. Although ORM libraries are designed to abstract away raw SQL and offer safer database interactions, improper usage – especially when dealing with dynamic or user-supplied input – can reintroduce classic security flaws.
In the following sections, we examine examples of both vulnerable and secure implementations across a range of popular technologies and frameworks, including:
- Hibernate (Java)
- SQLAlchemy (Python)
- Django ORM (Python)
- Entity Framework (C#/.NET)
- Sequelize (Node.js)
- Prisma (TypeScript/Node.js)
- Eloquent (PHP)
- Active Record (Ruby)
- GORM (Go)
These comparisons highlight common mistakes developers make and demonstrate how to properly leverage each framework’s security features to prevent SQL injection.
Hibernate (Java)
Hibernate is one of the most widely used ORM frameworks in the Java ecosystem. It abstracts away much of the boilerplate JDBC code and provides a convenient and type-safe way to interact with databases using Java objects. When used correctly, Hibernate offers strong protection against SQL injection — but unsafe query construction can still lead to vulnerabilities.
Vulnerable Implementation
String userInput = request.getParameter("userId");
String query = "from User where id = " + userInput;
List<User> users = session.createQuery(query).list();
Code language: JavaScript (javascript)
In this example, untrusted user input is directly concatenated into an HQL (Hibernate Query Language) statement. Since HQL queries are interpreted and translated into SQL by Hibernate, injecting malicious input such as 1 OR 1=1
can result in a query that returns all user records from the database. Even though HQL is more abstract than raw SQL, it remains vulnerable when used improperly — especially when queries are dynamically constructed with unsanitized user input.
Secure Implementation (Parameterized HQL)
String userInput = request.getParameter("userId");
String query = "from User where id = :userId";
List<User> users = session.createQuery(query)
.setParameter("userId", userInput)
.list();
Code language: JavaScript (javascript)
The secure version uses a named parameter (:userId
) and binds the value using setParameter()
. Hibernate automatically escapes and sanitizes the input when preparing the final SQL query for execution. This prevents attackers from injecting additional conditions or SQL logic into the query.
Full ORM-Based Implementation (Recommended)
String userInput = request.getParameter("userId");
Long id = Long.valueOf(userInput);
User user = session.get(User.class, id);
Code language: JavaScript (javascript)
This implementation utilizes Hibernate’s full ORM features. Instead of constructing HQL queries, the session.get
method is used to retrieve the User
entity by its primary key.
This approach is preferred for basic lookup operations, as it is concise, safe, and leverages the object-relational mapping model directly.
SQLAlchemy (Python)
SQLAlchemy is a popular Object-Relational Mapping (ORM) library for Python, widely used in web applications developed with frameworks such as Flask and Django. It provides a high-level API for communicating with databases while allowing developers to write SQL queries when needed. Despite its abstraction features, improper use of SQLAlchemy—especially when writing raw SQL queries—can still expose applications to SQL injection vulnerabilities.
Vulnerable Implementation
user_id = request.args.get('user_id')
query = f"SELECT * FROM users WHERE id = {user_id}"
result = db.engine.execute(query)
Code language: JavaScript (javascript)
User input (user_id
) is directly interpolated into a raw SQL string. If a malicious user supplies an input like 1 OR 1=1
, the resulting query becomes:
SELECT * FROM users WHERE id = 1 OR 1=1
This would return all users in the database, demonstrating a classic SQL injection attack. Since the query is built through string formatting, there is no mechanism to sanitize or escape user input, making the application highly vulnerable.
Secure Implementation
from sqlalchemy import text
user_id = request.args.get('user_id')
query = text("SELECT * FROM users WHERE id = :user_id")
result = db.engine.execute(query, user_id=user_id)
Code language: JavaScript (javascript)
The secure version uses SQLAlchemy’s text()
function, which creates a TextClause
object allowing for safe parameter binding. The :user_id
placeholder is automatically escaped and sanitized by SQLAlchemy, ensuring that even if the input is malicious, it won’t break out of the intended SQL structure.
Full ORM-Based Implementation (Recommended)
user_id = request.args.get('user_id')
user = db.session.query(User).filter_by(id=user_id).first()
Code language: JavaScript (javascript)
This implementation uses SQLAlchemy’s full ORM capabilities to retrieve a User
object directly from the database using object-oriented constructs. The query is built through SQLAlchemy’s query API, and parameters are automatically bound and escaped by the framework.
This method avoids raw SQL entirely, reduces the risk of injection, and aligns with the intended use of an ORM. It is the preferred approach for most standard CRUD operations in applications that use SQLAlchemy.
Django ORM (Python)
Django includes a powerful Object-Relational Mapper (ORM) that allows developers to interact with the database using high-level Python code. It encourages safe query construction through methods like .filter()
and .get()
, which internally use parameterized queries. However, security risks arise when developers fall back to raw SQL, especially using string interpolation.
Vulnerable Implementation
user_id = request.GET.get("user_id")
query = f"SELECT * FROM auth_user WHERE id = {user_id}"
users = User.objects.raw(query)
Code language: JavaScript (javascript)
In this example, the user_id
parameter is interpolated directly into a raw SQL query. Django’s raw()
method will execute the string as-is, so any malicious input can compromise the query and potentially lead to a SQL injection attack.
Secure Implementation
user_id = request.GET.get("user_id")
query = "SELECT * FROM auth_user WHERE id = %s"
users = User.objects.raw(query, [user_id])
Code language: JavaScript (javascript)
This version uses raw()
with bound parameters. Django safely escapes and binds the value, ensuring it’s treated as data and not part of the SQL structure. This is the correct way to use raw SQL in Django when necessary.
Even Better: Use the ORM’s QuerySet API
user_id = request.GET.get("user_id")
users = User.objects.filter(id=user_id)
Code language: JavaScript (javascript)
Django’s ORM methods like .filter()
automatically apply parameter binding behind the scenes.
This is the safest and most idiomatic way to fetch data in Django applications, and should always be preferred over raw SQL unless absolutely required.
Entity Framework (C#/.NET)
Entity Framework (EF) is Microsoft’s official Object-Relational Mapping (ORM) framework for .NET applications. It allows developers to interact with relational databases using strongly-typed .NET objects, and it supports LINQ (Language Integrated Query) for safe and readable data access. However, just like with any ORM, the use of raw SQL can introduce vulnerabilities if not handled carefully.
Vulnerable Implementation
string userName = Request.QueryString["name"];
var query = "SELECT * FROM Users WHERE UserName = '" + userName + "'";
var users = context.Database.SqlQuery<User>(query).ToList();
Code language: JavaScript (javascript)
The application retrieves a value from the query string and directly inserts it into a SQL query through string concatenation. If a user supplies a malicious input like ' OR '1'='1
the resulting query becomes:
SELECT * FROM Users WHERE UserName = '' OR '1'='1'
Code language: JavaScript (javascript)
This condition is always true, and it would return all records from the Users table — a classic SQL injection scenario. Despite using Entity Framework, this method completely bypasses its built-in protections by manually crafting the SQL query with unsanitized input.
Secure Implementation
string userName = Request.QueryString["name"];
var users = context.Users.Where(u => u.UserName == userName).ToList();
Code language: JavaScript (javascript)
This secure version leverages LINQ to Entities, allowing Entity Framework to construct a parameterized query behind the scenes. This means that even if the userName
input contains special characters or malicious content, it will be safely treated as a parameter value — not executable SQL.
Internally, EF converts this LINQ expression into a safe SQL query that looks like:
SELECT * FROM Users WHERE UserName = @p0
where @p0
is bound to the value of userName
. This binding automatically escapes characters and mitigates SQL injection risks.
Sequelize (Node.js)
Sequelize is a widely-used Object-Relational Mapping (ORM) library for Node.js, supporting various SQL dialects such as MySQL, PostgreSQL, SQLite, and MSSQL. It simplifies database interactions by allowing developers to work with JavaScript objects instead of raw SQL. When used properly, Sequelize offers built-in protection against SQL injection by supporting parameterized queries and query builders.
Vulnerable Implementation
const { Sequelize } = require('sequelize');
const sequelize = new Sequelize('database', 'username', 'password', {
host: 'localhost',
dialect: 'mysql'
});
const userInput = req.query.userId;
const query = `SELECT * FROM users WHERE id = ${userInput}`;
sequelize.query(query).then((results) => {
console.log(results);
});
Code language: JavaScript (javascript)
In this example, the userId
parameter from the query string is directly interpolated into the SQL string. If an attacker sends a request like:
/?userId=1 OR 1=1
The final query becomes:
SELECT * FROM users WHERE id = 1 OR 1=1
As we know, this condition always evaluates to true and can return all users in the table. The vulnerability arises because Sequelize’s query()
method executes the raw SQL string as-is when no parameterization is used, making the code susceptible to SQL injection attacks.
Secure Implementation
const { Sequelize } = require('sequelize');
const sequelize = new Sequelize('database', 'username', 'password', {
host: 'localhost',
dialect: 'mysql'
});
const userInput = req.query.userId;
const query = `SELECT * FROM users WHERE id = :userId`;
sequelize.query(query, {
replacements: { userId: userInput },
type: sequelize.QueryTypes.SELECT
}).then((results) => {
console.log(results);
});
Code language: JavaScript (javascript)
This secure version leverages parameterized queries using Sequelize’s replacements
option. The :userId
placeholder is automatically escaped and bound to the provided value, preventing malicious inputs from altering the structure of the query.
Internally, Sequelize translates this into a prepared statement, protecting the application against SQL injection.
Even Better: Use Sequelize Models
const { DataTypes } = require('sequelize');
const User = sequelize.define('User', {
id: {
type: DataTypes.INTEGER,
primaryKey: true
},
// other fields...
});
const userInput = req.query.userId;
User.findAll({
where: { id: userInput }
}).then((users) => {
console.log(users);
});
Code language: JavaScript (javascript)
By using Sequelize’s model abstraction, developers can interact with the database in a safe and expressive way. The .findAll()
method with a where
clause automatically handles parameterization behind the scenes.
This eliminates the risk of SQL injection entirely, while also making the code more readable and maintainable.
Prisma (TypeScript/Node.js)
Prisma is a modern ORM for Node.js and TypeScript, known for its developer-friendly API, strong typing, and default safety. It minimizes the risk of SQL injection by using a declarative syntax and avoiding raw SQL in most cases. However, when using raw queries via queryRaw()
, developers must be careful to avoid direct interpolation of user input.
Vulnerable Implementation
const userId = req.query.userId;
const users = await prisma.$queryRawUnsafe(`SELECT * FROM User WHERE id = ${userId}`);
Code language: JavaScript (javascript)
User input is directly interpolated into the query string using queryRawUnsafe()
. Prisma makes it explicit in the method name that this approach is dangerous. If untrusted input is used here, it can result in a SQL injection vulnerability.
Secure Implementation
const userId = req.query.userId;
const users = await prisma.$queryRaw`SELECT * FROM User WHERE id = ${userId}`;
Code language: JavaScript (javascript)
This version uses Prisma’s tagged template syntax, which automatically escapes and binds the parameters. Prisma ensures that the query is executed safely, treating the input as a value rather than executable code.
Even Better: Use Prisma’s Query API
const userId = req.query.userId;
const users = await prisma.user.findMany({
where: { id: Number(userId) }
});
Code language: JavaScript (javascript)
The preferred and most secure way to query data in Prisma is through its Query API. This method is entirely declarative and inherently safe from SQL injection, as it handles parameter binding internally and eliminates the need for manual SQL construction.
Eloquent (PHP)
Eloquent is Laravel’s default ORM, widely used in modern PHP applications. It provides a fluent, expressive syntax for interacting with databases using PHP models. When used properly, Eloquent offers built-in protections against SQL injection by abstracting query construction. However, manually writing raw SQL with user input can still introduce serious vulnerabilities.
Vulnerable Implementation
$userId = $_GET['userId'];
$users = DB::select("SELECT * FROM users WHERE id = $userId");
Code language: PHP (php)
In this example, user input is directly interpolated into a raw SQL query. Since there is no parameter binding or escaping, this code is vulnerable to SQL injection attacks. Even though Laravel provides a robust ORM, bypassing it with unsafe query construction undermines its security benefits.
Secure Implementation
$userId = $_GET['userId'];
$users = DB::select("SELECT * FROM users WHERE id = :id", ['id' => $userId]);
Code language: PHP (php)
This secure version uses parameterized queries. Laravel automatically escapes the bound value, ensuring the query remains safe regardless of the input.
Even Better: Use Eloquent Models
$userId = $_GET['userId'];
$users = User::where('id', $userId)->get();
Code language: PHP (php)
Using Eloquent’s query builder provides the highest level of abstraction and safety. The where()
method internally performs parameter binding, making it both secure and readable.
This approach is recommended for most use cases in Laravel applications.
Active Record (Ruby on Rails)
Active Record is the default ORM used in Ruby on Rails applications. It provides an intuitive and expressive syntax for interacting with relational databases using Ruby objects. While Active Record automatically uses parameterized queries under the hood, security issues can arise when developers fall back to raw SQL or unsafe interpolated strings.
Vulnerable Implementation
user_id = params[:user_id]
users = User.where("id = #{user_id}")
Code language: JavaScript (javascript)
User input is interpolated directly into the SQL string. If user_id
is not properly sanitized, this can lead to a SQL injection vulnerability. Such usage bypasses the built-in protections that Active Record normally provides.
Secure Implementation
user_id = params[:user_id]
users = User.where(id: user_id)
The secure version uses hash syntax to pass the parameter. Active Record automatically handles escaping and binds the value securely, preventing SQL injection.
When Raw SQL is Needed
user_id = params[:user_id]
users = User.find_by_sql(["SELECT * FROM users WHERE id = ?", user_id])
Code language: JavaScript (javascript)
If you must write raw SQL in Rails, use bound parameters in the form of array substitution. Active Record ensures the query remains safe by properly escaping the user input.
GORM (Go)
GORM is the most popular ORM library for the Go programming language. It provides a developer-friendly API for interacting with databases using Go structs. Although GORM promotes safe query construction by default, using raw SQL with untrusted input can still expose applications to SQL injection vulnerabilities.
Vulnerable Implementation
userId := c.Query("user_id")
query := fmt.Sprintf("SELECT * FROM users WHERE id = %s", userId)
var users []User
db.Raw(query).Scan(&users)
Code language: JavaScript (javascript)
User input is directly embedded into a raw SQL string using fmt.Sprintf()
. Since there is no parameter binding or escaping, this code is vulnerable to SQL injection if the user_id
parameter is manipulated.
Secure Implementation
userId := c.Query("user_id")
var users []User
db.Raw("SELECT * FROM users WHERE id = ?", userId).Scan(&users)
Code language: JavaScript (javascript)
The secure version uses GORM’s parameterized Raw()
query. The ?
placeholder safely binds the user input, ensuring that it is treated as data and not executable code.
Even Better: Use GORM’s Query Builder
userId := c.Query("user_id")
var users []User
db.Where("id = ?", userId).Find(&users)
Code language: JavaScript (javascript)
GORM’s query builder is the recommended approach for most use cases. It automatically escapes and binds parameters, greatly reducing the risk of SQL injection. Additionally, it produces cleaner and more maintainable code.
Best Practices for Preventing SQL Injection with ORM
To effectively guard against SQL injection when using ORM frameworks, it’s essential to combine proper coding techniques with security-conscious practices throughout the application stack. While ORMs are designed to reduce the risk of injection attacks, they are not foolproof — insecure usage can still expose applications to serious vulnerabilities. Below are key strategies to follow that help ensure your use of ORM remains both effective and secure.
Always Use Parameterized Queries
Parameterized queries remain the most reliable and recommended defense against SQL injection. Instead of embedding user input directly into SQL strings, these queries separate code from data, ensuring that inputs are treated strictly as values.
Most ORM frameworks offer built-in mechanisms for this:
- Hibernate: Use named parameters or positional placeholders.
- SQLAlchemy: Use the
text()
function with bound parameters. - Django ORM: Use the QuerySet API (
.filter()
,.get()
), or parameterized.raw()
queries. - Entity Framework: Use LINQ or
FromSqlInterpolated()
for safe raw SQL. - Sequelize: Use the
replacements
orbind
options. - Prisma: Use
$queryRaw
with tagged template syntax, or preferably the high-level Query API (findMany
,findUnique
). - Eloquent: Use placeholders in
DB::select
, orModel::where()
for safe queries. - Active Record: Use hash-style conditions or pass values as parameters.
- GORM: Use placeholders in
Raw()
queries orWhere(...)
with automatic parameter handling.
When raw SQL is absolutely necessary, never use string concatenation — always apply the ORM’s parameterization features.
Where possible, rely on your ORM’s built-in abstractions that apply these protections automatically.
Use ORM’s Built-in Security Features
Beyond parameterized queries, many ORMs provide higher-level abstractions that inherently enforce safe practices. These include query builders, model-based access patterns, and fluent APIs that handle parameter binding behind the scenes.
For example, methods like .where()
in Eloquent, .filter()
in Django, or findMany()
in Prisma safely handle user input by default. Similarly, LINQ in Entity Framework and criteria-based queries in Hibernate generate parameterized SQL automatically.
By leveraging these features, developers can write expressive, readable, and secure database queries—without having to manage input sanitization or SQL syntax manually.
Validate and Sanitize Input
Even with parameterization in place, input validation provides an important extra layer of defense. Ensure that user inputs conform to expected formats and constraints — for example, by checking the type, length, and value ranges or using allowlists for acceptable values.
Validation should be applied as early as possible in the request lifecycle, ideally before any interaction with the database or business logic occurs. This not only improves security but also helps catch user errors and avoid unnecessary processing.
Input validation is especially important when dealing with identifiers, search terms, numeric filters, or user-defined parameters that influence query structure. When combined with parameterized queries, it creates a strong foundation against injection, logic flaws, and unexpected behavior in application logic.
Keep ORM Frameworks Updated
ORM libraries, like any other dependencies, can have security issues—including vulnerabilities within the frameworks themselves. For example, Rails’ ActiveRecord, Sequelize, and node-mysql have had SQL injection vulnerabilities in certain versions, where the root cause was in the ORM framework rather than in the application code. This means that even when developers follow best practices, insecure versions of ORM tools can still expose applications to risk.
To reduce this risk, always keep ORM libraries up to date to benefit from bug fixes and security patches. Use tools like npm audit
, pip-audit
, or dotnet list package --vulnerable
to detect known vulnerabilities in your dependencies.
Additionally, consider integrating Software Composition Analysis (SCA) tools into your workflow. Tools like Snyk scan your dependencies using public vulnerability databases (e.g., NVD) and can alert you when a newly discovered CVE affects your project. They also provide remediation advice and integrate with CI/CD pipelines for continuous monitoring.
A secure implementation today could become vulnerable tomorrow if an underlying ORM framework vulnerability is discovered—so proactive dependency management is essential.
Implement Proper Access Controls
Even if a SQL injection occurs, limiting what the database user can do helps contain the damage. Applications should connect using database accounts with only the permissions they truly need — for example, read/write access to specific tables, but no rights to DROP
, ALTER
, or access sensitive administrative data.
Combined with other safeguards, access control helps reduce the blast radius of any vulnerability. This layered approach — incorporating parameterized queries, input validation, and least-privilege access — is essential for building secure applications.
Conclusion
ORM frameworks offer valuable abstraction and can help prevent SQL injection — but they’re not foolproof. Secure coding practices are still essential, especially when dealing with dynamic queries, raw SQL, or user-supplied input.
The examples across various technologies show how common mistakes can reintroduce vulnerabilities, even in applications that rely heavily on ORMs. At the same time, they highlight the power of built-in ORM features when used correctly.
To reduce the risk of SQL injection in ORM-based applications:
- Never concatenate user input directly into SQL
- Always use parameterized queries
- Validate and sanitize input
- Keep ORM libraries and dependencies up to date
- Apply the principle of least privilege for database access
By combining these practices, developers can build applications that are both productive and secure — without sacrificing flexibility or developer experience.
Thanks for reading — hope it was helpful!