Understanding and Mitigating TOCTOU Vulnerabilities in C# Applications
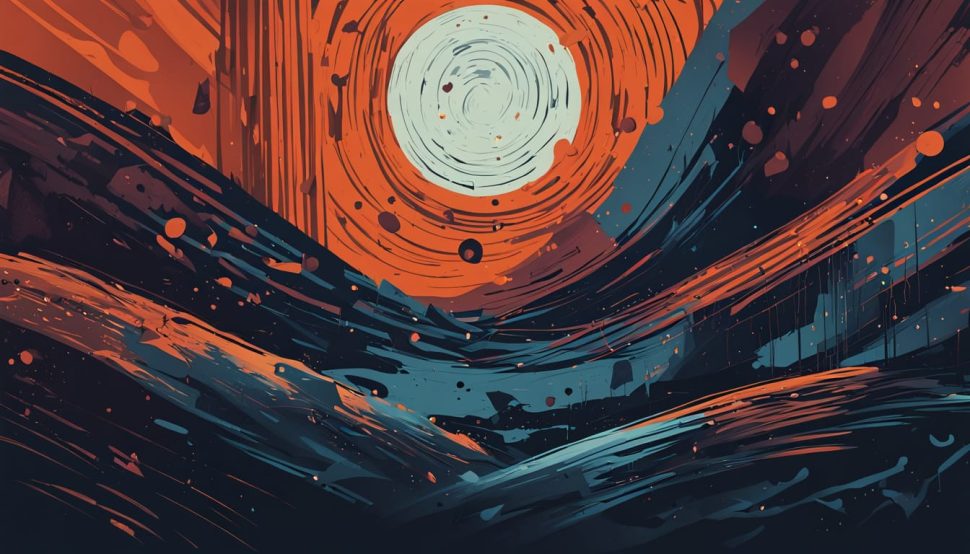
Time-of-Check to Time-of-Use (TOCTOU) vulnerabilities represent a critical class of race condition security flaws that plague software systems interacting with external resources. These vulnerabilities manifest when a program’s security check on a resource (such as a file, directory, or system object) becomes invalid before the resource is actually used, creating a window for attackers to manipulate system behavior.
In the context of C# development for Windows platforms, where file system operations and dynamic code loading are common, understanding TOCTOU risks becomes paramount for building secure applications.
The Anatomy of TOCTOU Vulnerabilities
Fundamental Mechanism
The TOCTOU vulnerability pattern follows a predictable sequence:
- Check Phase: The application verifies a resource’s properties, such as its existence, permissions, or contents.
- Race Window: An attacker modifies the resource between the check and its use.
- Use Phase: The application proceeds based on the original check, unaware of the modification.
This temporal gap between validation and utilization allows attackers to manipulate critical system resources.
In C# applications, TOCTOU vulnerabilities are commonly associated with file operations but extend to registry access, process handling, and dynamic code loading.
Windows-Specific Attack Surfaces
The Windows ecosystem introduces unique TOCTOU risks due to its design and file system behavior. Certain system features create opportunities for attackers to manipulate resources between validation and use:
- NTFS Junctions: Junctions (reparse points) allow attackers to redirect file operations to different locations in the file system, enabling privilege escalation or data manipulation. If an application checks the original file location but fails to track changes in its path, an attacker can swap the destination before the file is used.
- Transactional NTFS (TxF): Although largely deprecated, TxF allows file operations to be executed within a transaction. If an application checks a file’s state before use, an attacker can modify it within a transaction that remains invisible until committed. This enables the introduction of malicious modifications without detection.
- Handle Recycling: Windows aggressively recycles process and file handles. If an application improperly tracks handle validity, an attacker could forcefully close a legitimate handle and create a new object in its place, tricking the application into operating on a different resource than intended.
- Signature Validation Cache: Windows caches file signature validation results to improve performance. However, if an application checks a file’s signature before execution but fails to verify it at the time of use, an attacker could replace it with an unsigned or tampered file that exploits the cached validation.
- Assembly Loading: .NET’s ability to dynamically load assemblies introduces another TOCTOU attack surface. If an application checks an assembly’s integrity before loading but does not enforce security at the time of execution, an attacker may replace the assembly in the race window, leading to arbitrary code execution.
These attack surfaces illustrate how Windows-specific features can amplify TOCTOU risks, making it crucial for developers to adopt secure file and resource handling practices.
Mitigation strategies must focus on atomic operations, strong access control policies, and real-time validation at the moment of use rather than relying solely on pre-execution checks.
Common TOCTOU Patterns in C# Code
While TOCTOU is most commonly associated with file handling, similar vulnerabilities occur in dynamic code loading and process execution. Below are three examples that highlight different attack surfaces.
File Handling: Check-Then-Use Race Condition
One of the most common TOCTOU vulnerabilities in C# arises from sequential file checks that separate verification from access:
if (File.Exists(filePath))
{
// Race window exists here
var content = File.ReadAllText(filePath);
}
Code language: JavaScript (javascript)
In this scenario, an attacker could delete, modify, or replace the file between the File.Exists()
check and the File.ReadAllText()
operation, leading to unintended behavior, privilege escalation, or arbitrary code execution.
This issue is particularly dangerous when the file contains configuration settings, authentication tokens, or executable scripts.
Dynamic Code Loading: Swapping Assemblies
Another high-risk scenario occurs when an application verifies an external DLL before executing it:
Assembly LoadVulnerable(string path)
{
if (ValidateAssemblyHash(path)) // Check
{
// Race window: Attacker can replace the DLL before execution
return Assembly.LoadFrom(path); // Use
}
throw new InvalidOperationException("Assembly validation failed.");
}
Code language: JavaScript (javascript)
Since the assembly’s integrity is validated before loading, an attacker can swap the file between the check and execution.
This is particularly problematic in plugin-based systems or applications that load untrusted third-party libraries, as it can lead to the execution of malicious code.
Process Handling: Privileged Process Execution
TOCTOU vulnerabilities can also arise when checking for the existence or state of a process before interacting with it:
if (Process.GetProcessesByName("targetProcess").Length > 0)
{
// Race window: The process may terminate or be replaced
Process.Start("targetProcess"); // Unsafe assumption
}
Code language: PHP (php)
Between the check (GetProcessesByName()
) and the use (Process.Start()
), an attacker could terminate the original process and replace it with a malicious one.
If the application assumes that the process is safe based on the initial check, it may execute an untrusted binary with elevated privileges, leading to security breaches.
Defensive Programming Techniques
Mitigating Time-of-Check-Time-of-Use (TOCTOU) vulnerabilities requires adopting defensive programming techniques to ensure safe file access and reduce race conditions. These techniques focus on secure handle management, least privilege file access, and opportunistic lock (oplock) enforcement, which collectively help prevent attackers from exploiting file system race conditions.
Secure Handle Management
Instead of repeatedly closing and reopening file handles, a more secure approach is to maintain persistent handles throughout the file operation lifecycle. This prevents race conditions where a file’s state may be altered between operations. By keeping the handle open from validation through processing, developers can ensure that the file remains unchanged and prevent TOCTOU exploitation.
Example – Persistent File Handle Usage in C#:
using var fs = File.Open(path, FileMode.Open, FileAccess.Read, FileShare.Read);
ValidateFileContents(fs);
ProcessFile(fs); // Reuse the same file handle
Code language: JavaScript (javascript)
Least Privilege File Access
Following the principle of least privilege (PoLP) minimizes the risk of race conditions by restricting file-sharing permissions. When opening a file, developers should limit how other processes can access it during critical operations.
Example – Restricting File Access Using FileShare Modes:
var fs = new FileStream(
path,
FileMode.Open,
FileAccess.Read,
FileShare.Read | FileShare.Delete
);
Code language: JavaScript (javascript)
Oplock-Based File Protection
Opportunistic locks (Oplocks) are a Windows-based mechanism that allows a process to detect and respond to changes before another process modifies a file. This ensures that a file remains consistent throughout its entire lifecycle.
By using overlapped I/O and oplock mechanisms, applications can optimize file access by buffering operations and detecting when another process attempts to modify the file.
Example – Using Oplocks with Overlapped I/O in C#:
[DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
static extern SafeFileHandle CreateFile(
string lpFileName,
FileAccess dwDesiredAccess,
FileShare dwShareMode,
IntPtr lpSecurityAttributes,
FileMode dwCreationDisposition,
int dwFlagsAndAttributes,
IntPtr hTemplateFile
);
const int FILE_FLAG_OVERLAPPED = 0x40000000;
var handle = CreateFile(
path,
FileAccess.Read,
FileShare.Read,
IntPtr.Zero,
FileMode.Open,
FILE_FLAG_OVERLAPPED,
IntPtr.Zero
);
// Use oplock via overlapped I/O
Code language: JavaScript (javascript)
Using a combination of handle recycling, strict file permissions, and oplock mechanisms significantly reduces the risk of TOCTOU vulnerabilities.
By ensuring that files remain consistent throughout their processing lifecycle, developers can eliminate race conditions and prevent attackers from exploiting file system weaknesses.
Mitigation Strategies for C# Developers
C# developers can mitigate Time-of-Check-Time-of-Use (TOCTOU) vulnerabilities by ensuring uninterruptible file access, adopting thread-safe resource handling, leveraging secure file handling with file locks, and implementing atomic file operations.
These strategies help maintain file integrity, prevent race conditions, and enhance security against unintended modifications or exploitation.
Uninterruptible File Access
A common TOCTOU mistake occurs when developers use separate file existence checks (File.Exists(filePath)
) before opening a file, introducing a race condition where the file could be modified or replaced after the check. Instead of performing check-then-use operations, files should be opened in a single step, where any access errors are handled using exceptions.
try
{
using var fs = File.Open(filePath, FileMode.Open, FileAccess.Read, FileShare.Read);
var content = new StreamReader(fs).ReadToEnd();
}
catch (FileNotFoundException)
{
// Handle missing file
}
Code language: JavaScript (javascript)
By combining existence checks and file access in one atomic operation, this method eliminates race windows, preventing potential file modifications in between. The use of FileMode.Open
ensures that the operation will either succeed or fail instantly, without allowing an attacker time to intervene. If the file should be created if missing, FileMode.OpenOrCreate
can be used instead.
Why is
File.Exists()
unsafe? An attacker could replace the file between the existence check and file opening, leading to unauthorized modifications or execution of malicious content.
Thread-Safe Resource Handling
In multi-threaded applications, race conditions can occur within the same process, leading to unexpected file modifications or corruption. When multiple threads attempt to access the same resource, a lack of synchronization can result in inconsistent states or lost writes. Using thread synchronization mechanisms ensures that only one thread at a time can perform file operations.
private static readonly object _fileLock = new object();
void ThreadSafeWrite(string path, string data)
{
lock (_fileLock)
{
File.WriteAllText(path, data);
}
}
Code language: JavaScript (javascript)
This ensures that all write operations are serialized, preventing two threads from modifying the same file simultaneously.
However, this approach only protects against intra-process race conditions—if multiple processes attempt to modify a file concurrently, external locking mechanisms, such as file locks, should be used.
Secure File Handling with File Locks
For applications where multiple processes access the same file, race conditions can be mitigated using exclusive file locks. These locks prevent unauthorized modifications while the file is in use, ensuring that other processes cannot change its contents.
Using FileStream.Lock()
, a process can lock a file segment (or the entire file) to prevent modifications from external sources:
using var fs = new FileStream(path, FileMode.Open, FileAccess.ReadWrite, FileShare.None);
fs.Lock(0, fs.Length); // Lock the entire file
Code language: JavaScript (javascript)
This guarantees that no other process can modify or replace the file while it is open. Once the operation is complete, releasing the lock with fs.Unlock(0, fs.Length)
ensures that the file remains accessible for other processes. File locks are particularly useful when working with shared configurations, logs, or sensitive resources where consistency is critical.
Limitation:
FileStream.Lock()
does not prevent an attacker from renaming or deleting the file. UsingFileOptions.DeleteOnClose
can further mitigate these risks by ensuring that temporary files are cleaned up securely.
Atomic File Operations
TOCTOU (Time-of-Check to Time-of-Use) vulnerabilities occur when a file’s state is checked before being accessed, allowing an attacker to modify or replace the file between the check and its use. A more secure approach is to use atomic operations, ensuring that a file is either completely updated or remains unchanged.
Writing directly to a file introduces risks such as incomplete updates due to unexpected interruptions, which may lead to data corruption. To mitigate these risks, the safest approach is to write changes to a temporary file first and then atomically replace the original file. The File.Replace()
method guarantees that either the original or the new file exists, preventing an inconsistent state.
void AtomicWrite(string filePath, string newData)
{
string tempFile = Path.Combine(Path.GetDirectoryName(filePath), Path.GetRandomFileName());
// Securely create and write to the temp file
using (var tempFs = new FileStream(tempFile, FileMode.CreateNew, FileAccess.Write, FileShare.None))
using (var writer = new StreamWriter(tempFs))
{
writer.Write(newData);
writer.Flush();
tempFs.Flush();
}
// Atomically replace the original file
File.Replace(tempFile, filePath, null);
}
Code language: JavaScript (javascript)
This approach ensures that the file is never left in an incomplete state, maintaining data integrity. It also reduces race conditions that could allow unauthorized modifications before the replacement occurs, making the process more secure.
If preserving an older version is necessary, specifying a backup path in
File.Replace()
can ensure recoverability. This approach prevents accidental data loss and allows rollback in case of failure.
Detection and Prevention Tools
Proper detection and prevention mechanisms are essential to mitigate time-of-check-time-of-use (TOCTOU) vulnerabilities, particularly in file access operations. Various static and runtime analysis tools can help identify and prevent such issues before they become exploitable.
Below is an overview of commonly used tools and techniques.
Static Analysis
Static analysis tools help detect TOCTOU vulnerabilities at the code level before runtime. These tools analyze source code for patterns that indicate unsafe sequential checks and file operations.
- Klocwork SV.TOCTOU.FILE_ACCESS Checker: Flags instances where a file’s existence is checked before performing operations on it, helping to identify potential race conditions.
- Roslyn Analyzers: Custom static code analysis rules for detecting unsafe patterns related to
File.Exists
andFile.Open
in .NET applications, ensuring that developers follow secure coding practices. - CodeQL: A powerful static analysis framework that allows for writing custom queries to detect TOCTOU sequences in source code. It helps identify patterns where file state is checked and then used without proper synchronization.
While static analysis can catch many vulnerabilities at the source code level, it is not always sufficient, as it may miss race conditions that only manifest under specific timing or system conditions.
To complement static analysis, dynamic testing is necessary.
Runtime Protection
While static analysis is useful for preventing vulnerabilities before deployment, runtime protection mechanisms add an extra layer of security by detecting and mitigating TOCTOU vulnerabilities during execution:
- Handle Tracking: Monitors the lifecycle of file handles to prevent race conditions. By ensuring that file handles remain valid and unchanged throughout their use, this approach mitigates TOCTOU risks.
- File System Minifilters: Kernel-mode file system minifilters enforce operation locking (oplock) to prevent changes to files between the time of check and the time of use. This is particularly effective for preventing unauthorized modifications in critical system paths.
- CLR Instrumentation: By hooking into the .NET Common Language Runtime (CLR) assembly loading sequences, it is possible to monitor and restrict unsafe file access operations dynamically, ensuring security policies are enforced.
Comprehensive Security Testing
Beyond automated tools, regular security testing is essential for identifying TOCTOU vulnerabilities that may not be detected by static or runtime analysis alone. Organizations should incorporate the following testing methodologies into their security workflows:
- Manual Code Reviews: Security experts should conduct manual audits of high-risk file operations, privilege checks, and dynamic loading mechanisms to identify race conditions that automated tools might overlook.
- Fuzzing: TOCTOU vulnerabilities often depend on precise timing, making them difficult to detect through static analysis. Fuzzing techniques, such as forced file system manipulations and multi-threaded race condition testing, can help trigger potential exploits in real-world scenarios.
- Penetration Testing: Offensive security teams should conduct simulated attacks, attempting to exploit race conditions through file replacements, symbolic link attacks, and process injection techniques.
- Continuous Security Scanning: Integrating automated security tests into CI/CD pipelines enables earlier detection of TOCTOU in the development lifecycle, reducing remediation costs.
By leveraging a combination of static analysis, runtime protection, and active security testing, organizations can significantly reduce the risk of TOCTOU vulnerabilities and improve the overall security of their applications.
Ongoing security assessments ensure that new vulnerabilities are identified as applications evolve, reducing the likelihood of TOCTOU exploits in production environments.
Conclusion
TOCTOU vulnerabilities in C# applications represent a persistent security risk, particularly in environments that rely on file system operations, process management, and dynamic code execution. These flaws exploit race conditions that arise when applications check a resource’s state before using it, creating an opportunity for attackers to manipulate system behavior in the gap between verification and execution.
Mitigating TOCTOU requires a multifaceted security strategy that integrates secure coding practices, runtime protections, and continuous security assessments. Developers must prioritize atomic operations over sequential checks, enforce strict access controls, and incorporate cryptographic validation to ensure resource integrity. Additionally, security mechanisms such as handle tracking, process isolation, and file locking can significantly reduce the attack surface.
While technical mitigations are essential, security is an ongoing process. Proactive security testing, including static analysis, fuzzing, and penetration testing, helps uncover race conditions before they become exploitable. As new attack techniques emerge, staying informed about Windows security model improvements and best practices in secure development is critical to maintaining resilient applications.
By adopting a defense-in-depth approach, organizations can strengthen their applications against TOCTOU vulnerabilities while ensuring performance and reliability in modern .NET environments.